November 19, 2024
New React 19 Features You Should Know - Explained with Code Examples
React 19 was officially introduced in April 2024, bringing a wave of exciting features across React applications. By September 2024, there are 68,719,809 websites using React for their front-end frameworks with the USA alone accounting for 2,973,984 of them.

This new version of the 11-year-old JavaScript libraries is designed to simplify development, boost performance, and improve user experience for this huge user base.
In this article, Dirox will explore React 19’s standout features, with code examples to illustrate how they can enhance your development process and application performance.
1. Key Features of React 19
1.1. React Compiler
React 19's new compiler is its main attraction. By converting your React code to plain JavaScript, this compiler improves performance and, more importantly, eliminates the need for you to continuously adjust performance manually. This can save developers significant time and reduce the need for manual fine-tuning.
Code Example:
// No need for useCallback/useMemo
function Component() {
return <div>Optimized!</div>;
}
Although the compiler automates optimization, manual adjustments may still be necessary for high-performance applications, especially when managing complex data dependencies.
1.2. Server Components
Server Components in React 19 revolutionize rendering by enabling server-side processing for faster, more efficient user experiences. Key benefits include:
- Faster Initial Load Times: Reduces client-side JavaScript and starts data fetching on the server for quicker page loads.
- Improved Code Portability: Enables shared logic between server and client, reducing duplication and boosting maintainability.
- Enhanced SEO: Delivers pre-rendered HTML to improve search engine crawling and indexing.
Code Example:
// Users.server.jsx
// Server Component: Fetches data and returns JSX
export default async function Users() {
const res = await fetch("https://api.example.com/users");
const users = await res.json();
return (
<div>
<h1>Users</h1>
{users.map((user) => (
<div key={user.id}>
<h2>{user.name}</h2>
<p>{user.role}</p>
</div>
))}
</div>
);
}
Server Components in React 19 provide a middle ground between static site generation (SSG) and full client-side rendering.
Compared to older techniques like SSG, Server Components streamline content delivery by allowing developers to specify which parts of an app should be rendered server-side or client-side.
1.3. New use()
Hook
The new use()
hook simplifies asynchronous data handling, combining the functionality of useEffect, useState, and useContext. This can make managing data dependencies and complex states significantly easier.
Code Example:
import React, { use } from 'react';
// Function to fetch data
async function fetchData() {
const response = await fetch('https://api.example.com/data');
if (!response.ok) {
throw new Error('Failed to fetch data');
}
return response.json();
}
const DataFetchingComponent = () => {
// `use()` suspends the component until the promise resolves
const data = use(fetchData());
return (
<div>
<h1>Data:</h1>
<pre>{JSON.stringify(data, null, 2)}</pre>
</div>
);
};
export default DataFetchingComponent;
Here, use()
pauses rendering until a promise resolves, triggering a Suspense boundary if an error occurs. No useEffect was needed since React handles data fetching automatically, removing the need for manual side effects. Additionally, Suspense boundaries simplify error and loading state management, eliminating manual tracking.
use()
is particularly useful for context-dependent async operations, where multiple hooks were previously needed to manage loading states and data consistency.
1.4. Actions and Server Actions
React 19 introduces Actions for handling form submissions, asynchronous operations, and state management. Actions streamline form handling and enhance integration with React's concurrent features:
- Simplified Event Handling: Replace traditional handlers like onSubmit by passing FormData directly to action functions, eliminating manual parsing.
- Server Actions: Allow Client Components to call server-side functions directly, simplifying tasks like database queries or file system access without custom APIs.
Code Example:
async function action(formData) {
return await handleSubmit(formData);
}
In short, Actions simplify code, especially in high-concurrency applications, by managing async logic in a centralized way that aligns with server and client needs.
2. Advanced Features for Streamlined Development
2.1. Ref Handling as Props
React 19 allows refs to be passed directly as props without needing forwardRef, simplifying component composition.
Code Example:
function Child({ innerRef }) {
return <input ref={innerRef} />;
}
While this approach simplifies ref usage, passing refs as props could lead to side effects in complex applications, so developers should use caution in large projects.
2.2. Optimistic UI with useOptimistic
The useOptimistic hook simplifies optimistic UI updates during async data mutations:
- Instant Feedback: Render optimistic state immediately, showing users the expected outcome without delay.
- Auto State Reversion: React reverts to the original state if the update fails, maintaining data consistency.
- Enhanced UX: Immediate feedback makes the app more responsive and engaging.
Code Example:
const [optimisticState, setOptimistic] = useOptimistic(initialState);
These Optimistic UI updates are ideal for real-time applications, as they can significantly improve user experience by reducing perceived latency.
2.3. New Hooks for Form Handling
React 19 introduces useFormStatus and useFormState to streamline form handling, reducing boilerplate and simplifying state tracking:
- Access Parent Form Status: Works like a Context provider, enabling easy access to form state.
- Less Prop Drilling: Eliminates the need to pass form state through multiple props, streamlining component management.
- Simplifies Design Systems: Reduces boilerplate for components interacting with form state, focusing on common use cases.
Code Example:
const { pending } = useFormStatus();
return <button disabled={pending}>Submit</button>;
These hooks work seamlessly with external libraries and complex validation systems, making form management in React more robust.
2.4. Improved Asset Loading
React 19 includes new asset preloading APIs, such as prefetchDNS and preload, improving asset loading times.
These APIs can be optimized with HTTP/2 and CDNs, significantly improving performance for React applications distributed globally.
3. Cutting-Edge Features for Future-Proof Applications
3.1. Web Components Integration
React 19 simplifies the integration of Web Components, bridging the gap between frameworks and fostering modular development.
- Why It Matters: Web Components allow developers to create reusable, encapsulated components that work seamlessly across different frameworks and libraries. React 19's improved support ensures smooth interoperation, making it easier to include legacy Web Components in modern React apps.
- Transforming Legacy Systems: By integrating Web Components, organizations can modernize older applications without rewriting large chunks of code, creating a cost-effective path for digital transformation.
- Cross-Framework Compatibility: Developers can now build libraries that function consistently across various environments, boosting collaboration and code reusability.
3.2. Directives: use client and use server
React 19 introduces the use client and use server directives, providing clarity in distinguishing client-side and server-side code.
- Streamlined Server-Client Interactions: These directives enable better control over caching and asynchronous operations, improving performance and user experience.
- Managing Transitions: In large-scale applications, balancing client and server code becomes crucial. The new directives help developers manage transitions effectively, reducing the risk of errors and performance bottlenecks.
- Potential Pitfalls: While these tools enhance flexibility, developers must carefully manage dependencies between client and server components to avoid unnecessary complexity.
Code Example:
"use client";
function ClientComponent() {
return <div>Client Side</div>;
}
4. Additional Performance Enhancements
4.1. Hydration and Error Reporting
React 19 delivers significant improvements in hydration and error handling, streamlining the debugging process for server-client rendering issues.
- Smarter Hydration: Enhanced mechanisms address common hydration mismatches, ensuring a smoother user experience, especially in dynamic or interactive applications.
- Better Error Messaging: New error reporting tools provide more context and actionable insights, helping developers quickly diagnose and resolve issues in complex projects.
4.2. SEO and Metadata Management
React 19 simplifies managing document metadata like titles, descriptions, and meta tags with built-in support for Document Metadata, making SEO optimization easier and more efficient.
- Declarative Metadata with DocumentHead: The new DocumentHead component lets developers define metadata directly in their components, improving code organization and readability.
- Centralized SEO Management: Manage all SEO-relevant elements, such as titles and descriptions, in one place, streamlining updates and reducing complexity.
- Less Boilerplate: No more manual string manipulations or complex workarounds—DocumentHead enables concise, clean, and efficient metadata handling.
This feature not only saves time but also ensures React apps are more SEO-friendly out of the box.
Conclusion
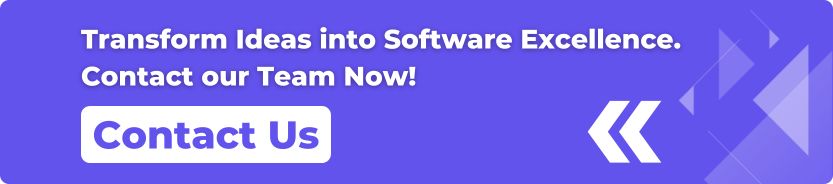
React 19 represents a leap forward in modern web development by equipping developers with the tools needed to build robust, future-proof applications. These new features are not just improvements—they set the stage for the next era of React development, emphasizing flexibility, interoperability, and performance.